Laboratory Training 2
C++ Operators and Statements
1 Training Tasks
1.1 Programmatic Implementation of Branching Algorithm
Develop a program that implements an algorithm for solving quadratic equation. The program should include checking all possible variants of the source data. In particular, the discriminant should be checked, and it should be checked whether the equation is quadratic. If the equation degenerates into a linear one, it is necessary to provide for finding the root of this linear equation, or to establish the presence of infinite count of solutions (absence of solutions).
1.2 Programmatic Implementation of Looping Algorithm
Develop a program that implements an algorithm for calculating the following expression:
Provide a check of possible errors.
1.3 Calculating Product
Write a program that reads x
and n
and calculates y
:
1.4 Calculating Sum
Write a program that reads eps
and calculates y
:
The loop terminates if new summand is less than eps
.
1.5 Individual Assignment
You should develop a program that calculates values of a function in a given range. The program should implement an algorithm developed in carrying out assignment 1.3 of previous lab.
2 Instructions
2.1 Main Elements of C++ Language
2.1.1 General Program Structure
The program in C++ consists of declarations and definitions of global names: types, constants, variables, and functions. In order to prevent name conflicts, the global scope is divided into namespaces.
In the global scope, you need to define the main()
function, which is starting point of your
program. All program code (except declarations and definitions) is allocated within functions.
In addition to the C++ text itself, the source code usually also contains preprocessor directives.
These directives start with the #
character and are processed by a special subprogram, the
so-called preprocessor. The minimum required directive is #include
. It causes the
inclusion text of another file (the so-called header file) in the current file of the. The header file
contains declarations and definitions needed to compile the source code. The header file name is specified in
angular parentheses (for standard files) or in quotation marks (for custom files in the current folder).
Physically, the text is not copied to the file, but the so-called translation unit is created in memory
– the source code processed by the preprocessor.
2.1.2 Identifiers. Keywords. Comments
Unlike Pascal and BASIC, C++ is case sensitive.
Java source code consists of tokens. Token is a sequence of characters that have some meaning. Separators (space, tab, new line, etc.) are placed between individual tokens.
Tokens are divided into the following groups:
- keywords (reserved words)
- identifiers
- literals (explicit constants)
- operators.
A keyword is a token that has a single predefined meaning in the program code. A set of keywords is finite. All keywords are reserved. Reserved words cannot be used as identifiers. There are 63 keywords in standard C++. Other 11 words are reserved for alternative representations of operators. Below is a list of keywords:
asm |
auto |
bool |
break |
case |
catch |
char |
class |
const |
const_cast |
continue |
default |
delete |
do |
double |
dynamic_cast |
else |
enum |
explicit |
export |
extern |
false |
float |
for |
friend |
goto |
if |
inline |
int |
long |
mutable |
namespace |
new |
operator |
private |
protected |
public |
register |
reinterpret_cast |
return |
short |
signed |
sizeof |
static |
static_cast |
struct |
switch |
template |
this |
throw |
true |
try |
typedef |
typeid |
typename |
union |
unsigned |
using |
virtual |
void |
volatile |
wchar_t |
while |
Identifiers used for naming variables, functions and other software objects. The first character must be a letter or underscore character ("_"). Numbers also can be used as non-starting characters. Using an underscore at the beginning of the name is not recommended since such names are often used in standard libraries.
It is advisable to use meaningful names that reflect the nature of the object or function. Do not use spaces inside identifiers. Therefore, if you want to create an identifier from several words, you can use one of two approaches:
- C style: use an underscore ("_") between words instead of a space
- C++ style: words are written together, starting the second, third and other words with a capital letter (camel notation).
For example, you can create the following variable names:
this_is_my_variable thisIsMyVariable
The C++ style is recommended.
Use English mnemonics for meaningful names. Names of user defined classes and structures should begin with capital letters, and other names – only with lowercase letters.
Comments are simply text that is ignored by the compiler. The comment is intended to inform the reader (programmer) about the meaning of types, objects and actions in the source code of the program. There are two types of comments in C++ language:
- The double-slash comment, which will be referred to as a C++-style comment, tells the compiler to ignore
everything that follows
//
, until the end of the line. - The slash-star comment mark (
/*
) tells the compiler to ignore everything that follows until it finds a star-slash (*/
) comment mark. These marks will be referred to as C-style comments. Every/*
must be matched with a closing*/
.
As a general rule, the large program should have comments at the beginning, telling you what the program does. Each large function should also have comments explaining what the function does and what values it returns.
2.2 Fundamental Data Types
Fundamental data types in C++ are divided into two categories:
- integer (whole numbers);
- floating (real numbers).
The group of integer types includes such types: int
, long
, short
,
char
, and bool
. All integer types, except for bool
,
come in two varieties: signed
and unsigned
. Integers without the word
unsigned
are assumed to be signed
. Signed integers are either negative
or positive. Unsigned integers are always positive. Character data (char
) are considered as
small integers.
According to the number of bits used to represent the data (range of values) we can speak about ordinary
integers (int
), short integers (short int
) and long integers (long
int
).
Floating point variables have values that can be expressed in fractions. In other words, they are real numbers.
The inner representation of floating point numbers allows to store fractional values in the form of significand*2exponent
.
This form provides the ability to store data in a very wide range.
The group of floating-point
types includes such types: float
, double
, and long
double
. The representation of long double
and double
is identical.
The following table shows size in bytes and range of fundamental types.
Category | Type Name | Another Name | Size in Bytes | Range of Values |
---|---|---|---|---|
Integer types | int |
signed int |
4 | -2 147 483 648 to 2 147 483 647 |
unsigned |
unsigned int |
4 | 0 to 4 294 967 295 | |
char |
signed char |
1 | -128 to 127 | |
unsigned char |
|
1 | 0 to 255 | |
short |
short int, signed short int |
2 | -32 768 to 32 767 | |
unsigned short |
unsigned short int |
2 | 0 to 65 535 | |
long |
long int, signed long int |
4 | same as int |
|
unsigned long |
unsigned long int |
4 | same as unsigned int |
|
bool |
|
1 | false or true (0 or 1) |
|
Floating point types | float |
|
4 | 3.4E +/- 38 (7 digits) |
double |
|
8 | 1.7E +/- 308 (15 digits) | |
long double |
|
8 | same as double |
A char
variable is commonly used to hold codes of characters. These small
integer values can be represented as characters. A character is a single letter, number, or another symbol.
Character variables hold a single byte and are used for holding the 256 characters and symbols of the ASCII and
extended ASCII character sets. ASCII stands for the American Standard Code for Information Interchange.
A variable of type bool
can have values true
and false
.
You can use one and zero instead of true
and false
.
2.3 Constant Values
Constants are tokens representing fixed numeric or character values, as well as a string of characters.
Integer constants can be decimal (base 10), octal (base 8) or hexadecimal (base 16). Decimal constants
must not use an initial zero. An integer constant that has an initial zero is interpreted as an octal constant.
All constants starting with 0x
(or 0X
) are taken to be hexadecimal. The suffix L (or
l) attached to any constant forces the constant to be represented as a long. Similarly, the suffix U (or u)
forces the constant to be unsigned. You can use both L and U suffixes on the same constant in any order or case:
ul
, lu
, UL
, and so on.
A character constant is formed by enclosing a single character from the character set within single quotation marks (' ').
Character combinations consisting of a backslash (\) followed by a letter or by a combination of digits are called "escape sequences". To represent a new line character, single quotation mark, or certain other characters in a character constant, you must use escape sequences. An escape sequence is regarded as a single character and is therefore valid as a character constant.
Escape sequences are typically used to specify actions such as carriage returns and tab movements on terminals and printers. They are also used to provide literal representations of nonprinting characters and characters that usually have special meanings, such as the double quotation mark ("). The following table lists the ANSI escape sequences and what they represent.
Escape Sequence | Represents |
---|---|
\a |
Bell (alert) |
\b |
Backspace |
\f |
Form feed |
\n |
New line |
\r |
Carriage return |
\t |
Horizontal tab |
\v |
Vertical tab |
\' |
Single quotation mark |
\" |
Double quotation mark |
\\ |
Backslash |
\? |
Literal question mark |
\ooo |
ASCII character in octal notation |
\xhh |
ASCII character in hexadecimal notation |
Constants of real types can be written in floating-point form or in exponential notation and have the
default type double
(double-precision floating-point number). If necessary, the
type of constant can be specified by writing the suffix f
or F
for the type
float
, the suffix l
or L
for the type long
double
. You can also explicitly use the suffixes d
and D
for
double
. For example:
1.5f // 1.5 of type float 2.5E-2d // 0.25 of type double
Negative floating constants are taken as positive constants with the unary operator minus (-
)
prefixed.
A string literal is a sequence of characters from the source character set enclosed in double
quotation marks (" "
). For example:
"This is the string"
The string constant may include control sequences. If there is nothing between the two constants except spaces and newline transitions, the compiler combines them into one. The string constant is represented by an array of characters containing, in addition to string characters, a final character with the code 0 ('\0'). Such strings are called null-terminated strings.
2.4 Defining Variables and Symbolic Constants
A variable is the named memory area that the program has access to. Each variable has a specific type. The variable is associated with its value, which is stored in a certain memory location (rvalue, right value, in the assignment is on the right), and its location, i.e. the address in memory, which stores its value (lvalue, left value).
The variable can be declared and must be defined. The syntax for declaring variables will be discussed below in the context of creating header files.
The definition of variable causes memory allocation. It starts with stating its type. The name of variable must be separated from type name by one or more space characters. The definition ends with a semicolon:
type_name variable_name;
It is advisable to use meaningful variable names. Variables with short names should have restricted scope.
If it is necessary to define several variables of the same type, their identifiers are written separated by commas:
int x, y; // Two integer variables with undefined values
Declarations and definitions are collectively called descriptions. It should be noted that when there
is no type name in the descriptions but there are other modifiers (long
,
short
, signed
, and
unsigned
), it is assumed that the variable has type
int
. For example,
short s; // short integer unsigned s; // unsigned integer
You assign a value to a variable by using the assignment operator (=). You would assign 5 to width
by writing
unsigned short width; width = 5;
You can combine definition with initialization:
unsigned short width = 5;
The initialization looks very much like assignment. The essential difference is that initialization takes place at the moment you create the variable.
There must be one and only one definition of a variable in the program. A variable cannot be used until it is defined. You can also initialize a variable with a constant in parentheses:
int a = 10; // An integer variable with an initial value of 10 int b(10); // The same
You can initialize more than one variable at creation. For example:
long width = 5, length = 7;
You can even mix definitions and initializations:
int myAge = 39, yourAge, hisAge = 40;
This example creates three type int variables, and it initializes the first and third.
In C++, we can assign integer values to floating-point variables and vise versa. Example:
double x = 10; int n = 10.5; // get integer part, n = 10
A symbolic constant is a constant that is represented by a name. Unlike a variable, however, after a constant is initialized, its value can't be changed. There are examples of defining constants:
const int yearOfBirth = 1901;
Here yearOfBirth
is a constant of type int
. Its value can't be changed.
If the type name was omitted during the definition, the constant is considered an integer. For instance:
const n = 2; // the same as const int n = 2;
This style of describing constants should be avoided.
In the 2011 version of C++ (C++ 11), an automatic type inference mechanism was
added to the language syntax, which allows the compiler to create local variables, which types depend on the
context.
To create these variables the auto
is used. These variables must be initialized. The variable type determines by compiler according to the type of
expression that is used for the initialization. Example:
auto i = 10; // integer variable auto x = 10.5; // variable of type double
Despite the fact that the type is not specified explicitly, the compiler creates a variable of the certain type. Once a variable is created, you cannot change its type.This approach only makes sense for complex types. This and other features of new versions of C++ will be discussed later.
2.5 Operators and Expressions
2.5.1 Expressions, Operators and Operands
An expression is a language construct that consists of one or more operations and can result in a specific result during program execution.
Operation is an atomic (indivisible) expression. The objects of operations are called operands.
An operator is a character (or several characters) that determines the action on the operands. Depending on the number of operands, there are unary operators (one operand), binary operators (two operands) and ternary operators (three operands).
2.5.2 Assignment
The assignment operator (=) causes the operand on the left side to have its value changed to the value on the right side. The expression
x = a + b;
assigns the value that is the result of adding a
and b
to the operand x
.
The assignment expression returns a value. After the assignment, an assignment expression has the value of the left operand. This value can be used by another assignment expression. For example
a = (b = (c = (d = 0))); // We assign 0 to all variables
In this example we don't need parentheses, because the assignment operator is right-associative:
a = b = c = d = 0;
2.5.3 Mathematical Operators
There are five mathematical operators: addition (+
), subtraction (-
),
multiplication (*
), division (/
), and modulus (%
). There are two ways of
division: floating point division and integer division. The first is everyday division. It works as you would
expect:
double a = 10; double b = 4; double c = a / b; // c = 2.5
The division operation on two integers gives an integer part of quotient:
int a = 10; int b = 4; int c = a / b; // c = 2 double d = a / b; // d = 2.0
Note. The result depends on types of operands. Compiler ignores type of a variable that obtains the result.
The modulus operator returns the remainder after an integer division:
int a = 10; int b = 3; int c = a % b; // c = 1
The both of operands must be integer.
Each operator has its own priority. Multiplication has a higher priority than addition. You can use parentheses to change the order of operations.
2.5.4 Self-Assignment Operators
The self-assignment operation can be represented as follows:
a op= b
In our case op
is an arithmetic or bitwise operator: + - * / % | & ^ << >>
. Each
self-assignment operation is equivalent to the following assignment:
a = (a) op (b);
For example,
x += 5;
equivalent to the expression
x = x + 5;
The self-assignment operation also returns a result: a new value for the variable.
2.5.5 Incrementing and Decrementing
Very often the value of an integer variable needs to be increased or decreased by one. Increment and decrement operators can be used instead of self-assignment:
k = 1; // Increment: ++k; // now k = 2, equivalent to k += 1 // Decrement: --k; // now again k = 1, equivalent to k -= 1
The increment and decrement operations have two forms: prefix and postfix. Prefix form provides an increase or decrease value of variable before this value will be used in expression, and postfix form modifies the value after its usage. For example:
k = 1; // Prefix form: n = ++k; // k = 2, n = 2 k = 1; // Postfix form: n = k++; // k = 2, n = 1
A similar example can be given for decrement.
2.5.6 Relational Operators
The relational operators determine equality and relative values of their operands. Every relational expression
evaluates to either 1 (true
) or 0 (false
). The table below shows
relational operators:
Name | Operator | Sample | Evaluates |
---|---|---|---|
Equals | ==
|
100 == 50; |
false |
50 == 50; |
true |
||
Not Equals | !=
|
100 != 50; |
true |
50 != 50; |
false |
||
Greater Than | >
|
100 > 50; |
true |
50 > 50; |
false |
||
Greater Than or Equals | >=
|
100 >= 50; |
true |
50 >= 50; |
true |
||
Less Than | <
|
100 < 50; |
false |
50 < 50; |
false |
||
Less Than or Equals | <=
|
100 <= 50; |
false |
50 <= 50; |
true |
Don't confuse the assignment operator (=
) with the equals relational operator (==
).
This is one of the most common C++ programming mistakes.
2.5.7 Logical Operators
The logical operators are used to combine multiple conditions. The table below shows logical operators:
Operation | Operator | Example |
---|---|---|
AND |
&& |
expression1 && expression2 |
OR |
|| |
expression1 || expression2 |
NOT |
! |
!expression |
A logical AND statement evaluates two expressions, and if both expressions are true, the logical AND statement is true as well. Otherwise, it evaluates false. A logical OR statement evaluates two expressions, and if both expressions are false, the logical OR statement is false. Otherwise, it evaluates true. A logical NOT statement evaluates true if the expression being tested is false. This statement evaluates false if the expression being tested is true.
Note: the logical AND is two &&
symbols and the logical OR is two ||
symbols. A single &
and |
symbols are so called bitwise operators.
Relational operators and logical operators have a precedence order. Relational operators have higher precedence than logical operators. It is often a good idea to use extra parentheses to clarify what you want to group.
2.5.8 The Comma Operator
The comma operator allows grouping two or more expressions. The value of the expression is the value of the last expression. The comma operator has left-to-right associatively. For example, the expression
x = (i = 0, j = i + 4, k = j);
equivalent to the expression
i = 0; j = i + 4; k = j; x = k;
The parentheses in this example are obligatory.
2.5.9 Conditional Operator
The conditional operator (?:
) is C++'s only ternary operator. It requires three
expressions and returns a value:
expression1 ? expression2 : expression3
This line is read as "If expression1
is true, return the value of expression2
;
otherwise, return the value of expression3
." Typically, the result would be assigned to a
variable. For example,
min = a < b ? a : b;
This statement assigns minimum value of a
and b
to variable min
.
2.5.10 The sizeof Operator
The sizeof
operator returns the size of its operand. The operand to sizeof
can be one of the following:
- A type name. To use
sizeof
with a type name, the name must be enclosed in parentheses. - An expression. When used with an expression,
sizeof
can be specified with or without the parentheses. The expression is not evaluated.
Example 3.1 demonstrates the use of the sizeof
operator.
2.5.11 Using Operators for Data Input and Output
There are no separate input and output operators in C++. The standard C library provides the
scanf()
and printf()
functions, which provide console input and output, respectively.
The use of these functions will be discussed later.
Instead of the scanf()
and printf()
functions, which do not provide proper type
control, C++ offers a more reliable and convenient way to input and output data. This method is based on the use
of I/O streams. C++ provides the ability to override operations for objects of standard and non-standard
classes. For the class that represents the input stream, a right shift operation (>>
) is
defined. The operator reads from the stream individual tokens and converts them into data that correspond to the
types of variables – operands, which are located to the left of the stream object. Similarly, a left shift
operation (<<
) for output is defined for the output stream. The output format is determined
by the types of variables. You can also display constants, in particular literals. To move the cursor to a new
line, you should put the endl
manipulator into the output stream.
In the following example, one integer, one valid value, and one character are read from the standard input stream (from the keyboard). The read values are displayed in a standard console window:
int k; double x; char c; cin >> k >> x >> c; cout << k << " " << x << " " << c << endl; // without spaces, the data will be joined together into one token
There are additional means for formatting data. For example, adding setw(n)
to the stream causes
the assignment of n
positions for output the current data.
To work with standard streams, you need to add an iostream
header file inclusion to the source
code. Tools for working with streams are defined in the std
namespace.
2.6 Statements
2.6.1 Overview. Null Statement
In C++, a statement is the simplest unit in a program code. Expressions and operations are parts in the statements. The sequence of statements implements a certain algorithm.
It is possible to offer the following classification of statements:
- description statements
- empty statement
- expression statements
- compound statement
- selection statements
- iteration statements
- jump statements
- exception handling statement.
Description statements are the only ones that can be located both inside functions and outside them. Description statements have been considered previously.
A null statement is just the semicolon and nothing else. A null statement is used when the statement is needed syntactically, but no action is required.
2.6.2 Expression Statement
All expressions with semicolon are expression statements. One of the most common statements is the following statement with assignment expression:
a = x + 1;
C++ allows you to create statements from operations that do not contain the assignment:
x + 1; a;
In most cases, such statements do not make sense, because the result of the operation is not stored anywhere.
But this is how functions with the result of type void
are called, for example:
system("pause");
Similar statements are also used for data input and output:
cin >> x; cout << x;
The following program calculates sum of two integer values entered by user:
#include <iostream> using namespace std; int main() { int a, b, c; cout << "Enter a and b: "; cin >> a >> b; c = a + b; cout << "Sum is " << c << endl; return 0; }
This program can be modified. The creation and calculation of c can be joined together:
... int a, b; cout << "Enter a and b: "; cin >> a >> b; int c = a + b; ...
The program can be more simplified. The creation of c variable has no sense. We can calculate sum immediately before its output. So final version will be as follows:
#include <iostream> using namespace std; int main() { int a, b; cout << "Enter a and b: "; cin >> a >> b; cout << "Sum is " << (a + b) << endl; return 0; }
Now compiler does not create extra cell in memory. Unnecessary copying also does not perform.
2.6.3 Compound Statement
A compound statement is a sequence of statements enclosed in braces {}
. A compound
statement is also called a block. The block does not end with a semicolon. Syntactically, the block can
be considered as a single statement. For example:
{ temp = a; a = b; b = temp; }
Compound statements are usually placed in those places of the program where the syntax allows the only statement, but the algorithm requires several actions.
The block is also important in determining the scope and lifetime of identifiers. The identifier declared inside the block has scope from the point of definition to the closing brace. The blocks can be nested indefinitely.
2.6.4 Selection Statements
Selection statements allow you to perform different actions depending on the conditions that arise during the execution of the program.
The condition statement (if
statement) controls conditional branching. The syntax for the
if
statement has two forms:
The first form:
if (expression) statement
The second form:
if (expression) statement1 else statement2
In the first form of the syntax, if expression is true
(nonzero), statement
is
executed. If expression is false
, statement
is ignored.
In the second form of syntax, which uses else
, the second statement is executed
if expression is false
. With both forms, control then passes from the if
statement to the next statement. The body of an if
statement is executed if the value of the
expression is not equal zero.
The statements (statement
, statement1
, statement2
) can be a single
statements ending with a semicolon or a blocks enclosed in braces. For example:
if (i > 0) y = x * i; else { x = i; y = f(x); }
In this example, the statement y = x * i
is executed if i
is greater than 0. If
i
is less than or equal to 0, i
is assigned to x
and f(x)
is
assigned to y
.
Note: good coding practice recommends always using a compound statement in
if
statements and loops, even if a single statement executed.
The algorithm for calculating the reciprocal value presented in the previous laboratory training can be programmatically implemented using a conditional statement:
#include <iostream> using namespace std; int main() { double x; cin >> x; if (x == 0) { cout << "Error" << endl; } else { double y = 1 / x; cout << y << endl; } return 0; }
Using if (x = 0)
instead of if
(x ==
0)
will not cause compiler errors, but will lead to unpleasant consequences.
As can be seen from the above example, in both branches of the if
statement there
are compound statements in accordance with good coding practice. In addition, the variable y is defined inside
the block in only one of the branches. This style is considered more correct than defining all variables in
advance.
The following program calculates the result of division:
#include <iostream> using namespace std; int main() { double a, b; cout << "Enter a and b: "; cin >> a >> b; if (a != 0) { double c = a / b; cout << "Quotient is " << c << endl; } else { cout << "Error" << endl; } return 0; }
The switch
statement allows for branching on multiple values of expression.
switch (expression) { /* body */ }
The switch statement body consists of a series of case
labels and an optional
default
label. The label consists of the case
keyword followed by a
constant expression. No two constant expressions in case
statements can evaluate to the same
value. The default
label can appear only once.
Execution of the switch consists of calculation of the control expression and transition to the group of
statements marked by a case
label which value is equal to the control expression.
If there is no such label, statements are followed after the default
label. During
the execution of the switch, the statements with the selected label are passed, and then the statements are
executed in the normal order. In order not to follow the statements that remain in the body of the switch, you
must use the break
statement. For example:
switch (i) { case 1: cout << "equals to 1"; break; case 2: cout << "equals to 2, "; default: cout << "not equals to 1"; }
The results of running previous code are following:
i | Output |
---|---|
1 | equals to 1 |
2 | equals to 2, not equals to 1 |
another | not equals to 1 |
The expression after switch
keyword must be integer.
2.6.5 Iteration Statements
Looping statements are presented in three versions:
- loop with a precondition,
- loop with a postcondition
- loop with a parameter.
The loop with the precondition is built according to the scheme:
while (condition) statement
A while
loop causes your program to repeat a statement as long as the starting condition
remains true
.
The loop with the postcondition is built according to the scheme:
do statement while (condition);
The statement is executed, and then condition is evaluated. If condition is true
, the loop
is repeated; otherwise, the loop ends. The do...while
loop executes the body of the loop
before its condition is tested and ensures that the body always executes at least one time.
The for
statement (loop with the parameter) is built according to the scheme:
for (expression1; expression2; expression3) statement
The for
loop implements the following algorithm:
- The
expression1
specifies the initialization for the loop. There is no restriction on the type ofexpression1
. - The
expression2
is evaluated before each iteration. If it is equal to zero, the transition to the next statement of the program, located after the body of the loop. - If
expression2
istrue
(nonzero), looping body (statement
) is executed - The
expression3
is evaluated. This expression is evaluated after each iteration. The process then begins again with the evaluation ofexpression2
.
The following examples demonstrate usage of loop statements for calculating the simple sum:
The while
loop:
int y = 0; int i = 1; while (i <= n) { y += i * i; i++; }
The do ... while
loop:
int y = 0; int i = 1; do { y += i * i; i++; } while (i <= n);
The for
loop:
int y = 0; for (int i = 1; i <= n; i++) y += i * i;
The for
loop is the most compact and reliable for calculating sums and products
with a fixed number of steps.
The statement
for( ; ; );
is the way to produce an infinite loop.
2.6.6 break, continue, and statements
In loops, break
terminates execution of the nearest enclosing loop statement. Control
passes to the statement that follows the terminated statement. A continue
statement forces
transfer of control to the controlling expression of the loop. Any remaining statements in the current iteration
are not executed. Most often break
and continue
are used in the
following constructions:
if (condition_of_early_end_of_loop) break; if (condition_of_early_end_of_iteration) continue;
The goto
statement allows you to go to the label. A label is an identifier with a colon in
front of the statement. The use of the goto
statement is in most cases impractical, as there
are structural structures, loops and blocks. The only case where the use of goto
is
reasonable is the interruption of several nested loops. For example:
int a; . . . double b = 0; for (int i = 0; i < 10; i++) { for (int j = 0; j < 10; j++) { if (i + j + a == 0) { goto label; } b += 1 / (i + j + a); } } label: // other statements
A label is some unique identifier followed by colon. In our example label is called label
.
3 Sample Programs
3.1 Size of Variable Types
The size of variable types:
#include <iostream> using namespace std; int main() { cout << "The size of an int is:\t\t" << sizeof(int) << " bytes.\n"; cout << "The size of a short int is:\t" << sizeof(short) << " bytes.\n"; cout << "The size of a long int is:\t" << sizeof(long) << " bytes.\n"; cout << "The size of a char is:\t\t" << sizeof(char) << " bytes.\n"; cout << "The size of a float is:\t\t" << sizeof(float) << " bytes.\n"; cout << "The size of a double is:\t" << sizeof(double) << " bytes.\n"; return 0; }
3.2 Character Codes
This program allows printing codes of given characters:
#include <iostream> using namespace std; int main() { char c; do { cin >> c; // Inputs character int i = c; // Converts char to int cout << i << '\n'; // Outputs code } while (c != 'A'); // until c == 'A' return 0; }
The do-while
statement executes a statement repeatedly until the specified termination
condition (the expression) evaluates to false
.
3.3 Hexadecimal Numbers
The following program prints hexadecimal representation of numbers:
#include <iostream> using namespace std; int main() { int i; do { cin >> i; // Inputs integer cout << dec << i << ' ' << hex << i << '\n'; } while (i != 0); // until i == 0 return 0; }
The state of the stream objects can be changed by using manipulators. You can set the base of the numbers for
display by using dec
(decimal), oct
(octal – base eight), or hex
(hexadecimal).
3.4 Integer Part of Values
It is necessary to write a program that prints integer part of floating numbers:
#include <iostream> using namespace std; int main() { double d; int i; do { cin >> d; // Inputs floating point value i = d; // Converts double to int cout << d << ' ' << i << '\n'; } while (i != 0); return 0; }
3.5 The if Statement and Conditional Operator
It is necessary write a program that reads x
and calculates y
x | y |
---|---|
less than -8 | 100 |
-8 to 1 | 200 |
greater than 1 | 300 |
3.5.1 Use of if Statement
#include <iostream> using namespace std; int main() { double x; cin >> x; double y; if (x < -8) { y = 100; } else { if (x <= 1) { y = 200; } else { y = 300; } } cout << y; return 0; }
Braces after if
and else
in this example are not
necessary, but their usage is recommended.
3.5.2 Use of Conditional Operators
#include <iostream> using namespace std; int main() { double x; cin >> x; double y = x < -8 ? 100 : (x <= 1 ? 200 : 300); cout << y; return 0; }
3.6 Linear Equation
The condition statement can be used for solving a linear equation:
In Example 3.1 of the previous laboratory training, a branching algorithm that solves this task was given. A program that implements this algorithm can be as follows:
#include <iostream> using namespace std; int main() { double a, b; cout << "Enter a and b: "; cin >> a >> b; if (a != 0) { double x = -b / a; cout << "Root is " << x << endl; } else { if (b == 0) { cout << "Infinite count of roots." << endl; } else { cout << "No roots." << endl; } } }
3.7 The switch Statement
It is necessary write a program that reads x
and n
and calculates
y
using a switch
statement:
n | y |
---|---|
1 | x |
2 | x2 |
3 | x3 |
4 | 1 / x |
other values | 0 |
#include <iostream> using namespace std; int main() { double x, y; int n; cin >> x >> n; switch (n) { case 1: y = x; break; case 2: y = x * x; break; case 3: y = x * x * x; break; case 4: y = 1 / x; break; default: y = 0; } cout << y; return 0 }
3.8 The Sum
It is necessary to write a program that reads x
and n
and calculates
y
:
The program must write error message if denominator equals zero.
#include <iostream> using namespace std; int main() { double x, y = 0; int i, n; cout << "Input x and n: "; cin >> x >> n; for (i = 1; i <= n; i++) { if (x == i) { cout << "Error!\n"; break; } y += 1/(x - i); } if (i > n) // Sum is calculated cout << "y = " << y << "\n"; return 0; }
Alternative solution:
#include <iostream> using namespace std; int main() { double x, y = 0; int i, n; cout << "Input x and n: "; cin >> x >> n; for (i = 1; i <= n; i++) { if (x == i) { cout << "Error!\n"; return 1; } y += 1/(x - i); } cout << "y = " << y << "\n"; return 0; }
3.9 The Product
It is necessary write a program that reads x
, k
, and n
and
calculates y
:
#include <iostream> using namespace std; int main() { double x, y = 1; int i, k, n; cout << "Input x, k, and n: "; cin >> x >> k >> n; for (i = 0; i <= n; i++) { if (i == k) { continue; } y *= (x + i); } cout << "y = " << y << "\n"; return 0; }
Alternative solution:
#include <iostream> using namespace std; int main() { double x, y = 1; int i, k, n; cout << "Input x, k, and n: "; cin >> x >> k >> n; for (i = 0; i <= n; i++) { if (i != k) { y *= (x + i); } } cout << "y = " << y << "\n"; return 0; }
3.10 A Product with Alternating Signs
In Example 3.3 of the previous laboratory training, the algorithm for calculating a product with alternating signs in multiplicands is given:
A program that implements this algorithm can be as follows:
#include <iostream> using namespace std; int main() { double x; int n; cout << "Enter x, n: "; cin >> x >> n; int k = 1; double p = 1; for (int i = 0; i <= n; i++) { p *= x + i * k; k = -k; } cout << "Product = " << p; return 0; }
3.11 The Exponent
Assume that we want to write a program that reads x
and calculates ex:
The loop terminates if new summand is less than 0.00001.
#include<iostream> using namespace std; int main() { double x, y = 0; double z = 1; // Summand int i = 1; cout << "Input x: "; cin >> x; while (z > 0.00001) { y += z; z *= x / i; i++; } cout << "y = " << y << "\n"; return 0; }
Alternative solution:
#include <iostream> using namespace std; int main() { double x, y = 0; double z = 1; // Summand cout << "Input x: "; cin >> x; for (int i = 1; z > 0.00001; i++) { y += z; z *= x / i; } cout << "y = " << y << "\n"; return 0; }
3.12 Nested Loops
In Example 3.4 of the previous laboratory training, the algorithm for calculating the sum of products is given:
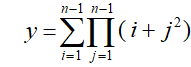
We'll implement calculations using nested loops. A program can be as follows:
#include <iostream> using namespace std; int main() { int n; cout << "Enter n: "; cin >> n; int sum = 0; for (int i = 1; i < n; i++) { int p = 1; for (int j = 1; j < n; j++) { p *= i + j * j; } sum += p; } cout << "Sum = " << sum; }
4 Exercises
Task 1
Write a program that reads characters and prints their hexadecimal code.
Task 2
Write a program that reads decimal integers and prints characters with appropriate codes.
Task 3
Write a program that reads floating point values and prints rounded values.
Task 4
Write a program that reads x
and calculates y
(signum function) using
an if
statement:
x | y |
---|---|
less than 0 | -1 |
0 | 0 |
greater than 0 | 1 |
Task 5
It is necessary write a program that reads x
and calculates a signum function using
conditional operators.
Task 6
Write a program that reads integer n
and calculates y
using a switch
statement:
n | y |
---|---|
0 | 2 |
1 | 4 |
2 | 5 |
3 | 3 |
4 | 1 |
other values | 0 |
5 Quiz
- What is the difference between C-style comment and C++-style comment?
- What is preprocessor?
- What is the difference between signed and unsigned integers?
- What is a constant?
- How to define hexadecimal constant?
- What is an escape sequence?
- What is a string literal?
- What is a variable?
- How to define a variable?
- What is a symbolic constant?
- How to use
sizeof
operator? - What is an expression?
- What is an operator?
- What is the difference between both assignment and self-assigned operators?
- What is the difference between prefix and postfix increment / decrement operators?
- To what type of values evaluates relational expression?
- What are logical operators?
- What is the "comma" operator?
- What is the conditional operator?
- What is a statement?
- What is an expression statement?
- What is the usage of the compound statement?
- What is the syntax for the
if
statement? - When we can use the
switch
statement? - How to use
break
statement withinswitch
statement? - What is the
default
statement? - What is the difference between precondition loop and postcondition loop?
- What are advantages of the
for
statement? - What is the difference between
break
andcontinue
statements?