Laboratory Training 1
Java Architecture and Syntax
1 Training Tasks
1.1 Individual Task
Create a console Java application that calculates values of a function on a given range. The necessary source data (interval boundaries and step of argument increasing) should be read from keyboard.
Program should contain reading data from keyboard and main loop, in which the following activities are performed:
- calculating the function choosing one of branches, depending on the value of the argument
- output to the console argument and result at each step
- increase value of the argument by the size of the step and go to the next calculation, if necessary.
Program should contain the single class with two static functions:
- a separate static function to calculate the value of y depending on the value of the argument x;
- the
main()
method, which contains the reading the source data, as well as the cycle of calculating the function and output the values of x and y.
Particular function is given in the individual task according to your own index in the group students list (index
of variant). Define the constant n with the final
modifier. Use the printf()
function
for formatted output.
1.2 Powers of 8
Read integer value of n
(from 1 to 10) and display powers of 8 from 1 to n. Implement two approaches:
using arithmetic and bitwise operations.
1.3 Use break and continue with a Label
Enter the values of x and n, calculate and display the result of the expression:
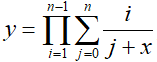
If i is equal to j + x, go to the next step of the external cycle without multiplying by the
intermediate result. To proceed to the next step, use continue
with a label.
If the denominator at any iteration is 0, exit both loops with an error message. To exit, use break
with
a label.
1.4 Type Conversion Function Library
Create a class with static functions to convert an argument of type boolean
to an
integer value (0 or 1) and vice versa (zero is false
, non-zero value is true
).
Demonstrate the invocation of functions by calling them from another class created in another package. Apply normal
and static import.
2 Instructions
2.1 Java Programming Language and Java Platform
2.1.1 Overview
Java as software term can be understood in two ways:
- programming language
- software platform
Work on the Java language began in 1990. Sun Microsystems programmer Patrick Naughton formulated the problems of adapting software to different platforms and devices that existed in the company. Patrick Naughton was included in an autonomous group led by James Gosling. The first name of this language was Oak. The name Java appeared in 1995.
At the same time, the global Internet emerged and developed rapidly in the world. In 1995, the company introduced its own HotJava browser with applet support.
On January 23, 1996, the official release of JDK 1.0 was released.
In 1997, Microsoft tried to include support for substantially modified Java in Visual Studio 97. After a lawsuit in 2001, Microsoft lost the right to continue using the Java trademark.
Java has the following main features:
- cross-platform (syntax and a set of standard tools not depend on a particular operating system and type of computer)
- standardized
- object-oriented
- strong typed (limited number of primitive types, strong rules of type conversion)
- easy to learn and develop
- provides increased reliability due to a lack of pointers and strict checking of classes that are loaded, and actions performed by the program
- adapted for Internet programming
To avoid many common mistakes inherent to C++ programs, the mechanism of automatic garbage collection is offered. This mechanism includes automatic counting of references to an object Java. When the object is no longer referenced, it is considered unnecessary and can be deleted from the memory, freeing up resources for the program.
Java is similar to C++. However, Java is cross-platform, uses bytecode interpreter, uses a different object model (similar to C#), that is, another set of object-oriented concepts and a set of class description tools and relationships between them, as well as Mechanisms for creating objects, etc.
Unlike many other languages and programming systems, the Java source code is not compiled directly into machine instructions, but into the so-called bytecode. The command set of this code must be interpreted to run on a specific computer running a particular operating system. In other words, we need a special software platform.
The software platform generally includes a set of software that provides the execution of other programs. Software tools that make the software platform, as a rule, have a standard set of features, technically different implemented on different hardware platforms and operating systems. Java-platform is made up of Java virtual machine and standard classes.
Java Virtual Machine (JVM) is a program that interprets and executes Java bytecode previously created from source code by Java compiler.
In addition to the Java language, the following languages are also designed to develop Java applications:
|
|
|
In addition, a number of previously created universal languages also provide the creation of applications for the JVM:
|
|
|
The number of languages targeted at the JVM is expanding very rapidly. New languages with support for JVM are emerging.
Conceptually, the Java platform is implemented at two levels, depending on the purpose of software applications:
- Java Card is a technology that allows you to safely run small Java applications (applets) on smart cards and similar small memory devices.
- Java ME (Micro Edition) - defines subset of libraries for devices with disabilities; it is often used to develop applications for mobile devices, PDAs, TVs and printers.
- Java Platform, Standard Edition, (Java SE)
-
standard edition of Java platform, designed to create and run applications for individual use. - Java Platform, Enterprise Edition, or Java EE, is API and runtime environment for developing and running enterprise software.
Technically, Java SE platform also implemented at two levels (up to and including Java 8):
- Java Runtime Environment (JRE): Java Virtual Machine with standard class libraries.
- Java Development Kit (JDK): software means for Java development
.
It includes Java compiler (javac
), standard Java class libraries, examples, documentation, as well as (JRE); both sets are distributed free of charge.
2.1.2 Java Versions
Each new Java version extends features of previous one.
- Java version 1.2 (December 4, 1998) was so much better than platform 1.1 that starting with it, all subsequent versions were called the Java 2 platform.
- Starting with JDK 1.5 (September 29, 2004) we speak about Java 5. This version significantly expands the syntax and tools of standard libraries.
- The version of Java 6 (JDK 1.6, December 11, 2006) differs from the previous one mainly by the extended class libraries.
- The version of Java 7 (July 28, 2011), in addition to the extended class libraries, provide new convenient syntax structures.
- Java 8 (March 18, 2014) thanks to the expansion of interface capabilities, the presence of lambda expressions, as well as the emergence of the Stream API provides the ability to implement functional and declarative programming. This significantly affected the style and expressiveness of Java programming.
- Starting in 2017, Java versions are added every six months.
Among the latest versions, it is possible to single out versions with long-term support, which, instead of other versions, are planned to be supported for several years, but not until the publication of the next version. The latest version with long-term support is Java 21 (September 2023). Further examples in the text are oriented towards using Java 21.
Unfortunately, in the versions starting from the ninth, the principle of backward compatibility is not fully implemented. Code created in previous versions of Java cannot be executed without further processing and recompilation. Because of these issues, Java 8 remains a common version among developers and is still supported by Oracle (until December 2030).
2.1.3 Installing Java 21
In order for Java application can be run on a particular computer, you must install JDK. On the oracle.com website, you go Java downloads (Products | Java | Download Java). You can immediately go to the page https://www.oracle.com/java/technologies/downloads/. Among the available versions you will choose the one for which long -term support is carried out. Today this is Java 21.
Choose the installation option for our operating system (Installer). If you use Windows operating system, x64 Installer will be the best option. After downloading the installer, you are offered a folder for the location of the application (can be left unchanged). Close button should be pressed on the last page of the installation wizard.
After completion, you can check the success of the installation by typing in the command prompt (Start |
Run... cmd
)
the following command:
java -version
The JRE version will be displayed in the console window.
2.2 IntelliJ IDEA Integrated Development Environment
2.2.1 IDEs for Java Programming
In the early years of Java, programmers used compilation tools that were started from the command line. However, for other programming languages there were powerful development environments, such as Visual C++, Borland Delphi and others. Visual means of environments to automate the creation and implementation of programs significantly increased the productivity of programmers. In total, there are dozens of commercial and free IDEs for Java. The following are the most popular environments, as well as those that have historically had the greatest impact on the development of Java development.
- Historically, the first was Visual Café for Java (1996) - an environment that was distributed on a commercial basis. The project existed until 2002.
- The release of the commercial environment Microsoft Visual Studio 97 (1997) included support for Visual J++ - "Java from Microsoft". Then Visual J# was supported as a .NET development language (until 2007).
- JBuilder is a commercial environment created in 1997 by Borland. The latest version of CodeGear JBuilder 2008 was released in 2009.
- Oracle JDeveloper is a free development environment (since 2005), the first version of the 1998 IDE was commercial. The environment is primarily focused on the use of Oracle technology. The latest stable version was released in 2016.
- BlueJ is an open source Java development environment used by beginners to learn programming, as well as in small projects.
- NetBeans is a free development environment for several programming languages (primarily Java). The NetBeans IDE project is supported and sponsored by Oracle. NetBeans successfully competes with the most common environments (Eclipse and IntelliJ IDEA), but is inferior in flexibility and performance.
- Eclipse IDE is the world's most popular cross-platform free development environment. Eclipse was originally developed by IBM as the successor to the IBM VisualAge development environment. Eclipse is characterized by flexibility and the ability to increase functionality through the plug-in mechanism.
- JetBrains IntelliJ IDEA is the most powerful environment for professional development of Java applications. The IDE is distributed in two versions. The free version of Community Edition allows you to create Java SE applications, the full version of Ultimate Edition, which allows, in particular, developing server solutions, is commercial.
2.2.2 Installing the IntelliJ IDEA IDE and Creating the First Project
The IntelliJ IDEA integrated development environment is an application written entirely in Java. However, Java does not need to be pre-installed on the computer to install the IDE. When installing IntelliJ IDEA, a special version of the Java virtual machine - JetBrainsRuntime - is automatically installed. But for compiling applications, JetBrainsRuntime cannot be applied. The standard JDK version must be installed.
There are two IDE variants that support different subsets of Java technologies. The free version (Community Edition) provides a complete set of development tools for the Java SE platform in Java, Kotlin, Groovy and Scala, project management tools, working with repository, testing and debugging. Ultimate Edition supports a full range of technologies, including Java EE, Spring Framework, special database tools, support for JavaScript and TypeScript development, and more.
The IntelliJ IDEA software installer can be downloaded from the JetBrains Download IntelliJ IDEA - The Leading Java and Kotlin IDE page. Choose the Ultimate Edition option.
Note: you can also download the Community Edition from this page, but students with academic accounts can use the full version (Ultimate Edition).
After downloading the installer, you need to run it. Clicking the Next button, go through the pages of the installation wizard.
- On the Choose Install Location page, select the folder to install (you can leave it unchanged).
- On the Installation Options page, leave other options unchanged.
- On the Choose Start Menu Folder page, select a group from the Start menu and click Install.
- After installing IntelliJ IDEA on the last page of the wizard, you can immediately select the option Run IntelliJ IDEA and click Finish.
After the first launch of the environment, you should accept the terms of the IntelliJ IDEA User Agreement. If IntelliJ IDEA was previously installed on the computer, you are offered a window in which you can choose to import the settings of the previous version. If you are installing IntelliJ IDEA for the first time, you do not need to import any options.
After the first launch of the environment, you should you are offered a window in which you can choose to import the settings of the previous version. If you are installing IntelliJ IDEA for the first time, you do not need to import any options.
To activate IntelliJ IDEA, you can use an academic account by clicking the Log In to JetBrains Account... button. A browser window will open in which you can either log in to an existing JetBrtains account or create a new one. Next, in the Licenses window, you can click the Activate and Continue buttons.
In the Welcome to IntelliJ IDEA window, the Projects position is selected by default. It is advisable to start from this position at the first start. Choose the creation of a new project (New Project button).
Note. In the Welcome to IntelliJ IDEA window you can make general settings for environment styles. The color theme chosen at will. You can also change the font size, keymap, etc.
On the new project wizard most used options are chosen by default (e.g. Java).IntelliJ IDEA is trying to find the installed JDK. If this fails, you can select the Add JDK... function and specify the path to the previously installed JDK, or install the JDK by downloading it from the Internet (Download JDK function).
If you select the Add sample code option and do not select the Generate code with onboarding tips option, the Main.java file with the following code will be added to the project:
public class Main { public static void main(String[] args) { System.out.println("Hello world!"); } }
In the body of the main()
function, you can place the required program code instead of output of
"Hello World" string.
To run the program for execution, you can use the Run | Run 'Main' main menu function, the green arrow in the toolbar or the Shift+F10 keyboard shortcut. If the compilation is successful, the program will execute. At the bottom of the main window there is a special area that simulates the work in the command window.
If you enable the Generate code with onboarding tips option when creating a new project, more complex source code will be generated. Working with this code allows you to try more complex ways of working, e.g. debugging, using a hint to fix individual pieces of code, etc.
2.2.3 Elements of the IDE IntelliJ IDEA Graphical User Interface. Code Templates and Hotkeys
The main IDE window includes both traditional for all modern integrated environments UI elements (menu, toolbar, main editor window, status bar) and IntelliJ IDEA-specific set of tool windows. Tool windows are associated with call buttons located around the perimeter of the workspace, with an icon, signature and numerical designation (last not necessarily). If you click on these buttons, windows with some auxiliary functionality will open next to them.
There is no save function in the standard main menu. Autosave occurs at program run or exit.
The submenus of the main menu (Navigate, Code, Analyze, and Refactor) offer powerful code management tools. Some functions for working with the code and the corresponding keyboard shortcuts are given in a table:
Menu Function | Description | Keyboard shortcut |
---|---|---|
Navigate | Back | Go to the previous view or editing place | Ctrl+Alt+Left |
Navigate | Forward | Go to the next view or editing place | Ctrl+Alt+Right |
Navigate | Previous Highlighted Error | Go to the previous error found in the code | Shift+F2 |
Navigate | Next Highlighted Error | Go to the next error found in the code | F2 |
Code | Override methods | Override the base class method | Ctrl+O |
Code | Implement methods | Implement an abstract class or interface method | Ctrl+I |
Code | Generate... | Generate code snippet (constructor, getters and setters, overridden methods, etc.) | Alt+Insert |
The IntelliJ IDEA editor supports a large number of keyboard shortcuts for editing. In addition to standard buffer operation, it allows you to delete a line (Ctrl-Y), to duplicate a line or block (Ctrl-D), go to breakpoints (Ctrl+breakpoint_number), comment and uncomment a block (Ctrl+/), and so on.
The Ctrl-space shortcut allows you to get a list of possible object items, method parameters, and so on.
The Ctrl-Shift-space
key shortcut filters the list, leaving only the variants of the expected type.
Using Ctrl+Alt+L, you can format the code.
A useful Alt-Enter keyboard shortcut allows you to get a set of options to fix an error that has occurred (for example, add an import directive, generate an empty method, etc.).
A list of all keyboard shortcuts can be found in the Settings window, then Keymap (File | Settings...) .
The line immediately after the menu bar shows the path to the file we are editing (inside the project), as well as the most commonly used functions (selecting a program to run, start and debug buttons, getting the project structure, and search).
The IntelliJ IDEA environment makes it much easier to type source code using Live Templates. You can call up the corresponding function via the main menu (Code | Insert Live Template...) or by pressing Ctrl-J. A list of code templates appears. The list depends on the context (location of the cursor in the editor window). The most useful code templates are given in a table:
Sequence of Characters | Program Code |
---|---|
psvm |
public static void main(String[] args) |
St |
String |
psf |
public static final |
fori |
for (int i = 0; i < ; i++) |
itar |
for (int i = 0; i < args.length; i++) { String arg = args[i]; } |
ifn |
if ( == null) { } |
iter |
for (Object o : ) { } |
sout |
System.out.println(); |
Templates can also simply be typed in code with the appropriate sequence of letters. After the prompt window appears, confirm the entry and get the corresponding text in the editor window.
2.2.4 Using Debugger
The debugger program can be started using the Run | Debug 'Main' main menu function, using button with the green bug in the toolbar or using the keyboard shortcut Shift+F9. In the simplest case, to debug the program, simply specify the breakpoint (click on the vertical gray bar to the left of the desired line) and start the debug process. The execution of the program will stop at the selected line, after which the intermediate values of the variables can be seen in the Variables output area or simply by placing the mouse cursor over the variable in the program code. In the Run | Debugging Actions submenu you can choose various versions of the stepwise program running:
- Step into (F7) – stepwise execution of called functions
- Step over (F8) – stepwise execution without entering called functions.
2.2.5 Project Structure
A separate folder is created for each project (with the name of the project, e. g.
Project
).
The project root folder files contain information about the project as a whole and about the modules (the
project may include several separate modules).
The src
folder contains source code packages of the project. The out
folder contains
the result of compiling the source code. Inside the out
folder is the production
subdirectory with Project
subfolder in it, then the folder structure will repeat the similar folder
structure of the src
subdirectory.
Additional project options related to compilation, encoding, etc. are allocated in the hidden
.idea
subfolder.
2.3 Java Fundamentals
2.3.1 Common Program Structure. Program Execution
There are no global variables in Java. That should avoid name conflicts. Neither are global functions nor procedures,
for that matter. There are only classes with fields (data members) and methods (member functions). A program in
Java consists of one or more class definitions. Some Java classes can have public
modifier.
One of public classes should define a method called main()
. This is the point at which the program
starts running. A source code of a class definition should be stored in text file with the .java extension.
Class name (without extension) must match the name of a public class that defined in the file. Please keep in mind
that capitalized and small letters are different.
Each class is compiled into its own file containing the binary code and .class
extension. Unlike C++,
this binary code is not a set of machine commands of particular processor, but so-called byte code.
Compiled program can be executed on your computer only if so-called Java Virtual Machine is installed. Java Virtual Machine (JVM) is a special program, which provides interpreting of byte codes. This approach allows you to create applications that can be transferred from one operating environment (operating system, hardware platform) to another without additional recompilation. Unlike C++, Java does not provide static linking of binary code into an executable file. Linking (getting of a byte code from different files with the .class extension and interpreting of byte code) is always dynamic.
Starting with Java 9, a modular code structure is proposed. A special file module-info.java
that
can be created in the project contains such information:
- definition of modules on which this module depends,
- information about the packages that this module exports,
- list of services provided by the module.
The modular structure increases the efficiency of loading and executing large Java applications.
2.3.2 Identifiers, keywords, and reserved words. Comments
Like C++, Java language is case-sensitive.
Java source code consists of tokens. Token is a sequence of characters that have some meaning. Separators (space, tab, new line, etc.) are placed between individual tokens.
Tokens are divided into the following groups:
- keywords (reserved words)
- identifiers
- literals (explicit constants)
- operators.
A set of keywords is finite. All keywords are reserved. Keywords cannot be used as identifiers. In Java there
are also reserved words that are not keywords. They generally cannot be used. These words are
const
and goto
.
Note: starting with Java 10, context-sensitive keywords such as var
or record
(in Java 14) have appeared in the language; these words are not
reserved, but have a definite meaning in a certain context.
Identifiers are used for naming variables, functions and other software objects. The first character must be a letter or underscore character ("_"). Numbers also can be used as non-starting characters. Using an underscore at the beginning of the name is not desirable.
It is advisable to use meaningful names that reflect the nature of the object or function. Do not use spaces inside identifiers. So if you need to create identifier with several words, these words should be written down together, starting second, third and other words with capital letters (the so-called camel notation). For example, you can create a variable name:
thisIsMyVariable
Use English mnemonics for meaningful names. Names of classes and interfaces should begin with capital letters,
and other names - only with lowercase letters. Names of static constants are composed of capital letters and an
underscore characters, for example PI
, CONST_VALUE
etc.
The Java language supports three kinds of comments:
- C++-style (double slashes)
- C-style (
/* */
) - Javadoc comments (
/** */
).
The JDK javadoc
tool uses doc comments when preparing automatically generated documentation. The
doc comments must be formatted according to Javadoc standards. You can add class and interface-level Javadoc
comments as well as method, constructor and field-level comments. Doc comments must be placed before corresponding
parts of code. Each comment consists of a description followed by one or more tags. If desired, you can use HTML
formatting in your Javadoc comments. There is a special set of Javadoc tags such as @author
,
@param
, @return
, and so on. The tags allow standard representation of documentation
concerning classes and methods. For example, the following comments before method
/** * Returns file name with given index.<br> * File names are stored in array of strings. * @param i index * @return file name. * */ public String getFileName(int i) { return names[i]; }
produce such fragment of HTML documentation file:
Method Detail |
getFileName
public String getFileName(int i)
- Returns file name with given index.
File names are stored in array of strings.
- Parameters:
i
- index- Returns:
- file name.
Note: in order to get a Javadoc comment template in IntelliJ IDEA, you should enter the comment start
(/**
) before necessary peace of code; the required template will be generated automatically.
2.3.3 Definition of Local Variables. Primitive Types
Java supports local variables, declared within method definitions. These local variables behave just like local variables in C++. Here are examples of local variables definition.
int i = 11; double d = 0, x; float f; int j, k;
You can define and initialize local variables anywhere in a function body or other block of code. You cannot use the same variable names in outer and inner blocks:
{ int i = 0; { int j = 1; // OK. j is local variable defined in inner block int i = 2; // Error! A variable i is defined in outer block } }
You cannot declare variables without definition.
The final
keyword can be applied to variables. Such variables cannot be modified.
final int h = 0;
The const
keyword is a reserved word in Java. This word cannot be used in any way.
Primitive, or basic, data types are classified as integer (for whole numbers), floating-point (for decimal
numbers), character (for Unicode characters), or Boolean (specifying true
/ false
).
The definition of local variables of primitive types looks like one in C++ programming language. The following
table shows a set of primitive data types in Java.
Keyword | Description | Size |
---|---|---|
Integers | ||
byte |
Byte-length integer (from - 128 to 127) | 8-bit |
short |
Short integer (from - 32768 to 32767) | 16-bit |
int |
Integer (from - 2147483648 to 2147483647) | 32-bit |
long |
Long integer (from -9223372036854775808 to 9223372036854775807) | 64-bit |
Real numbers | ||
float |
Single-precision floating point | 32-bit |
double |
Double-precision floating point | 64-bit |
Other Types | ||
char |
A single Unicode character | 16-bit |
boolean |
A Boolean value (true or false ) |
For some languages (for instance, C and C++), the format and size of primitive data types may depend on the platform on which a program runs. In contrast, the Java programming language specifies the size and format of its primitive data types for all platforms.
Integer constants are represented by a sequence of decimal digits. The default type of such constants is
int
. It can be adjusted by adding letter L
or l
(long
type) at the end
of the constant. Integer constants can be represented in octal notation, in this case the first digit should be
zero (0), and the number can only contain digits 0...7. Integer constants can also be represented in hexadecimal
number system, in this case constant starts with 0x
(or 0X
) characters. To represent
digits between 10 and 15, letters a
, b
, c
, d
, e
, and
f
(large or small) are used. For example:
int octal = 023; // 19 int hex = 0xEF; // 239
Java 7 also provides the ability to define binary constants (using prefixes 0B
or 0b
):
int m = 0b110011; // 51
In addition, groups of digits in constants can be separated by an underscore character, for example: int
n = 1_048_576;
A literal character value is any single Unicode character between single quote marks. You can use whether symbols of the current character set, or integer constant, which precedes the backslash character. It is a set of special control characters (these double characters are called escape sequences):
'\n' - a new line, '\t' - horizontal tab, '\r' - jump at the beginning of line, '\'' - single quote, '\"' - double quote, '\\' - backslash character itself.
Java uses Unicode table to store character type data in memory.
Constants of real types can be written whether with decimal point or in scientific notation and are double
by default. If necessary, type of constant can be specified by adding suffix f
or F
for
type float
, d
or D
for type double
. For example:
1.5f // 1.5 (float) 2.4E-2d // 0.024 (double)
You cannot assign floating point values to integer variables. A narrowing conversion - converting from a larger
type (for instance, double
) to a smaller type (for instance, float
) -
risks data. A narrowing conversion requires a special operation called a cast. For example
int i = 10; float f = i; // Permissible conversion long l = f; // Error! Narrowing conversion long l1 = (long) f; // Type cast
You can specify a long integer by putting an 'L'
or 'l'
after the number.
'L'
is preferred as it cannot be confused with the digit '1'
.
Numeric literals without decimal point are constants of type int
. Numeric literals with
decimal point are constants of type double
. To assign them to smaller types, you must use type cast:
float f = 10.5; // Error! Narrowing conversion float f1 = (float) 10.5; // Type cast float f2 = 10.5f; // Clarification of type. No errors
The Java programming language has no unsigned data types.
The two Boolean literals are simply true
and false
. There is no conversion
between the boolean
type and other types (specifically int
). You cannot
apply explicit typecasting for obtaining int
from boolean
and vice
versa.
The string constant is a series of characters enclosed in quotation marks. For example:
"This is the string"
Adding strings to variables of other types provides conversion of values into their string representation. In particular, this approach is used to output values of several variables. For example:
int k = 1; double d = 2.5; System.out.println(k + " " + d); // 1 2.5
Java 10 (and later versions) allows you to create implicitly typed local variables. To define such
variable, you should use context-sensitive keyword var
. These variables must be initialized.
The variable type determined by compiler according to type of initialization expression. For example:
var i = 1; var s = "Hello";
Despite the fact that the type is not specified explicitly, the compiler creates a variable of particular type. Once a variable is created, you cannot change its type:
var k = 1; k = "Hello"; // Error!
2.3.4 Expressions and Operations
Java class includes fields (data) and methods (functions) Methods may contain statements, which in turn are composed of expressions. Expression consists of one or more operations. Objects of operations are called operands. Operations can be unary (one operand), binary (two operands) and ternary (three operands).
Java supports almost all the standard numeric, logical and comparison C++ operators. These standard operators have the same precedence and associativity in Java as they do in C++. The addition and subtraction is less priority than multiplication and division, assignment is less priority than arithmetic operations, etc. To change the order of operations, use brackets.
Arithmetic operations include +
, -
(binary and unary), *
, /
,
as well as the operation to get the remainder from division %
(applied to integers only). If / is applied
to integers, the result of the division will also be integer, and the remainder will be discarded. If at least one
operand of a floating point type (real), you get a real result.
System.out.println(1 / 2); // 0 System.out.println(1.0 / 2); // 0.5
Relational operations include checking for equality ==
and for inequality !=
,
as well as >
(greater), >=
(greater or equals), <
(less), and <=
(less
or equal). These operations always produce value of type boolean
.
Logical operations include logical AND (&&
), logical OR (||
),
and logical NOT (!
). Instead of &&
and ||
, you can use bitwise &
and |
.
In this case, the full calculation of the values of both operands is always complete, while calculating the value
of the second operand of the operations &&
and ||
may not be implemented if the
result is already clear. These operations also produce value of type boolean
.
Assignment operations include simple and compound assignment. The result of the simple assignment operation is the value of the expression assigned to the left-hand operand. Compound assignment can be represented in general the following way:
a op= b
In this case, op
is an arithmetic or a byte operation: + - * / % | & ^ << >>
.
Each compound operation is equivalent to assignment:
a = (a) op (b);
For example,
x += 5;
which is equivalent
x = x + 5;
The increment operation (++
) provides an increase of a variable by one.
It has two prefix and postfix forms. The prefix form provides an increase of the variable before its value is used,
and postfix - after. The decrement operation (--
) decreases a variable by one. The rules for
its use are similar.
Java supports the comma operator only in loop headings. For example:
int i, j; for (i = 0, j = 0; i < 10; i++, j += 2) { System.out.println(i + " " + j); }
Use of bitwise operations &
(bitwise AND), |
(bitwise
OR), ^
(exclusive OR), ~
(bitwise NOT), <<
and (bitwise shift) similar
to C++. The usage of >>
operator assumes keeping number sign. Java provides additional unsigned
shift operator (>>>
).
Conditional (ternary) operation has the following form:
condition ? expression1 : expression2
First, the condition
value is calculated. If it is true
,
then the expression1
is calculated and its value is returned as a result of the conditional operation.
If the condition value is false
, then the expression2
is calculated and
its value is returned. The following example calculates the minimum of two numbers::
min = a < b ? a : b;
Java does not allow direct work with the pointers, therefore, there are no dereferencing operations,
getting item by the pointer and taking the address (*
, ->
and &
).
Java does not support the sizeof()
operation because its main purpose in
C++ is to find out the size of data when transferring source code to another platform. In Java, sizes of all data
types are standardized.
2.3.5 Statements. Control over Program Execution
Statements are the smallest separate parts of the programming language. A program is a sequence of statements. Most of Java statements are similar to ones in C++.
Empty statement consists of one semicolon.
Expression statement is an expression that ends with a semicolon. For examples are as follows:
k = i + j + 1; // assignment Arrays.sort(a); // invocation of a function return; // termination of current function execution
Unlike C++, expressions that don't make sense, such as
i + j + 1;
lead to compiling error.
Compound statement is a sequence of statements enclosed in braces. Compound statement is often referred to as block. Compound statement does not contain semicolon after closing brace. Syntactically block can be considered as a separate statement, but it also defines scope of visibility. Identifier declared inside the block, has a scope from the point of definition to the closing brace. Blocks can be nested in each other.
Selection statement is whether conventional statement or switch statement. Conditional statement is used in two forms:
if (condition_expression) statement1 else statement2
or
if (condition_expression) statement1
If condition_expression
is true
then statement1
is
executed, otherwise flow of control jumps to statement2
(in first form), or to the next statement (in
second form).
Unlike C++, expression-condition can only be of type boolean
. Therefore,
the following code
int k; // ... getting k if (k) { // check that k is not zero }
leads to compiling error. You should write
if (k != 0) { }
The switch allows you to select one of several possible branches of computation and is constructed according to the scheme:
switch (integer_expression) block
The block is as follows:
{ case constant_1: statements case constant-2: statements ... default: statements }
The implementation of the switch consists in the calculation of the control expression and the
transition to a group of statements marked with a case-label, the value of which is equal to the control expression.
If there is no such label, flow of control jumps to the statements after the default
label (which
may be also missing). When the switch is executed, all statements after the selected label are executed in the normal
order. In order not to execute the statements remaining in the body of the switch, you must use the break
statement.
Java allows usage of string expressions in switch
()
statement.
In this case, the constants (following case) should also be strings, such as:
case "some text":
Starting with Java 12, in addition to switch
statement, you can use switch
operation.
Common switch
operation syntax is as follows:
Type1 variable = switch (expression) { case VALUE1 -> expression1OfType1; case VALUE2 -> expression2OfType1; ... default -> expressionNOfType1; // this branch can be missing };
The switch
operation can also be used as part of a more complex expression.
The use of switch
operation allows you to reduce the source code and make it more readable.
Suppose, depending on the contents of some string ("one" or "two"), you should put to the variable n
the
corresponding integer, or 0 otherwise. The traditional code (switch
statement) will be as follows:
String number; // ... int n; switch (number) { case "one": n = 1; break; case "two": n = 2; break; default: n = 0; }
With switch
operation, the required value can be obtained in this way:
String number; // ... int n = switch (number) { case "one" -> 1; case "two" -> 2; default -> 0; };
Starting with Java 13, instead of ->
operator, you can use the yield
keyword.
The cyclic statements are implemented in three forms: a loop with precondition, a loop with postcondition and a loop with parameter (there is the fourth form concerned with arrays and collections).
A loop with precondition is constructed according to the scheme:
while (condition_expression) statement
At each iteration of the cycle, the condition expression of Boolean type is calculated and if
the value of this expression is true
, the body of the cycle (statement) is executed.
A loop with postcondition is constructed according to the scheme:
do statement while (condition_expression);
The expression-condition of Boolean type is calculated and verified after each repetition of the body, the loop is repeated until the condition is satisfied. The statement is performed at least once.
The cycle with the parameter is constructed according to the scheme:
for (E1; E2; E3) statement
Here E1
, E2
, and E3
are expressions of appropriate types.
A cycle with a parameter is implemented by such an algorithm:
- the expression
E1
is calculated (usually this expression performs preparation before the beginning of the cycle); - the Boolean expression
E2
is calculated and if it is equal tofalse
, the transition to the next statement of the program (after the cycle) is performed; - if
E2
istrue
the body of the cycle is executed; - the expression
E3
is calculated: preparation for repetition of the cycle is performed, after which the expressionE2
again is executed.
The following examples demonstrate usage of loop statements for calculating the simple sum:
y = 12 + 22 + 32 + ... + n2
The while
loop:
int y = 0; int i = 1; while (i <= n) { y += i * i; i++; }
The do ... while
loop:
int y = 0; int i = 1; do { y += i * i; i++; } while (i <= n);
The for
loop:
int y = 0; for (int i = 1; i <= n; i++) y += i * i;
Cycles can be put into one another.
In conjunction with the cycle statements, the jump statements are used: the break
statement,
which allows interrupting the execution of the innermost of the loops, the continue
statement,
which interrupts the current iteration of the innermost of the while
, do
,
or for
loops. Often, break is used in the following design:
if (early_termination_condition) break;
The break
and continue
statements, used alone, behave the same in Java
as they do in C++. However, in Java, they may optionally be followed by a label that specifies an enclosing
loop.
The labeled forms of these statements allow you to "break" and "continue" any specified loop
within a method definition, not only the nearest enclosing loop. For example:
int a; . . . double b = 0; label: for (int i = 0; i < 10; i++) { for (int j = 0; j < 10; j++) { if (i + j + a == 0) { break label; } b += 1.0 / (i + j + a); } }
The goto
statement is not currently part of the Java language, but goto
is
a reserved word in Java. This word cannot be used in any way.
There is no typedef
operator in Java. Java does not support C++ struct
and union
types.
2.4 Packages and Functions
Java code does not require header files. To avoid possible naming conflicts a mechanism of packages is used. In the Java language, packages are used to group classes.
A package is a collection of related classes and other providing access protection and namespace management. In the Java language, every class should be placed into a package. Physically each package is in fact a folder with the same name as package. Subpackages are reflected to subfolders.
The first line of most Java programs (not taking into account blank lines and comments) is so called package
statement:
package package_name;
If the source code for a class doesn't have a package statement at the top, declaring the package the class is in, then the class is in the default package. Classes and other syntactic structures of this package cannot be accessed from the outside. This option is not desirable.
It is desirable to use more meaningful package names. You can create nested packages, respectively, by creating
subfolders. In addition, it is desirable to ensure the uniqueness of package names. You can use the reverse
domain
name to ensure uniqueness. For example, if the domain name is author.com
, then you should use the
com
package,
then the author
subpackage and then the subpackages, according to different tasks. The following
examples
will use the ua.inf.iwanoff
package, which is the inverse of the author's domain name. This package
will be used for creation subpackages.
Java does not allow creation of global functions. Instead of this, you can create so-called static methods within classes. In simplest case, definition of a static method can be as follows:
static result_type function_name(list_of_formal_parameters) body
Unlike C++, Java does not allow creation of function prototypes (function declaration without definition).
Parameters of a function defined in brackets are called formal parameters. Parameters, which are used by invocation of a function, are called actual parameters (arguments). The storage for formal parameters is allocated by invocation of a function. Appropriate cells are created in the call stack. Values of actual parameters are copied into these cells.
Function's body is a block (compound statement). In the following example, function returns sum of two arguments:
static int sum(int a, int b) { int c = a + b; return c; }
You can implement this function without c
variable:
static int sum(int a, int b) { return a + b; }
To invoke function from another block, you should use its name followed by actual arguments (constants, variables, or expressions of appropriate types):
int x = 4; int y = 5; int z = sum(a, b); int t = sum(1, 3);
It can be no parameters in argument list:
int zero() { return 0; }
The call of such function also requires empty brackets:
System.out.println(zero());
The return
statement within function body causes termination of function's execution. The
expression
after return
keyword defines a value, which will be return from this function.
Sometimes, function returns no value. To declare such function, void
keyword is used as
resulting
type.
void hello() { System.out.println("Hello!"); }
In this case, you can omit return
keyword in function's body. The
return
statement
(without succeeding expression) allows termination of function before end of body. Functions with
void
type
can be called using separate statement only:
hello();
Parameters are passed to functions by value, that is, the values of actual arguments are copied to the memory allocated for the formal parameters. In this case, the values with which the function works are its own local copies of the actual parameters and their change does not affect these parameters:
static void f(int k) { k++; // k = 2; } public static void main(String[] args) { int k = 1; f(k); System.out.println(k); // k = 1; }
Unlike many other programming languages, Java does not provide a mechanism for passing parameters of value types by reference.
Within a single class, you can create several functions with the same names. Such functions must have different argument lists (count and/or types of arguments). Such approach is called function overloading. If names and argument lists are the same but resulting types are different, compiler produces an error.
Function overloading is used when you want to apply analogous operations to different types using different algorithms:
static int max(int a, int b) { } // Finding of maximum of two integers static int max(double x, double y, double z) { } // Finding of maximum of three floating point values
In order to determine what function should be called, the number and types of actual parameters specified in the call are compared with the number and types of formal parameters of all descriptions of functions with the given name. As a result, a function whose formal parameters are best matched to the call parameters is called, or an error occurs if no such function found.
In contrast to C++, you cannot create functions with default arguments.
Static functions within the class are called using only the name of the function and the list of actual
parameters.
In order to call the static function of another class, it is necessary to specify its name followed by the
function
name. The dot character is used as a separator. This way you can refer to the names within the package, as well
as to the classes and functions of the java.lang
package. This package contains classes with very
useful
features. For example, the Math
class provides a large number of mathematical functions.
Function | Meaning | Example |
---|---|---|
double pow(double a, double b) |
Calculation of ab | Math .pow(x, y) |
double sqrt(double a) |
Calculation of square root | Math .sqrt(x) |
double sin(double a) |
Calculation of sine | Math .sin(x) |
double cos(double a) |
Calculation of cosine | Math .cos(x) |
double tan(double a) |
Calculation of tangent | Math .tan(x) |
double asin(double a) |
Calculation of arc sine | Math .asin(x) |
double acos(double a) |
Calculation of arc cosine | Math .acos(x) |
double atan(double a) |
Calculation of arc tangent | Math .atan(x) |
double exp(double a) |
Calculation of ex | Math .exp(x) |
double log(double a) |
Calculation of natural logarithm (base e) | Math .log(x) |
double abs(double a) int abs(int a) |
Obtaining of the absolute value | Math .abs(x) |
long round(double a) |
Obtaining of the closest integer value | Math .round(x) |
In addition to mathematical functions, the Math
class provides such useful constants as Math.PI
,
Math.E
.
In order to access classes, which are defined in other packages (not in java.lang
), you should use
special prefix:
package_name.class_name
. If you want to access classes of nested
packages,
prefix will be more complex. To avoid use of full names, import
directive is used. The
import
directive
should be placed at the top of source file (or directly after package
statement).
There are three ways to import a class or an interface from another package:
- Import the class or the interface (
import
package_name.imported_name
). - Import the entire package of which the class or the interface is a member (using
import
keyword). - Static import (import of static elements of the specified class)
The following example demonstrates two of these ways.
import java.io.FileReader; // Import of the class or the interface import java.util.*; // Import of the entire package public class TestClass { public static void main(String[] args) { java.io.FileWriter fw; // Fully qualified name FileReader fr; // Direct access to the imported name; ArrayList al; // ArrayList is a part of in java.util package . . . } }
You never need an import
java.lang.*
statement to use the compiled classes in
that package. This package is always imported automatically.
A new additional form of import that was added in Java 5 version, allows import of static element of a given
class.
Most programs that need numerical functions of Math
class contain the following statement:
import static java.lang.Math.*;
Now you can invoke static methods of Math
class without extra qualifiers:
double d = sin(1);
2.5 Console Input and Output
Java provides several options for console input:
- direct use of
System.in
input stream, in particular itsread()
function; - use of
java.io.Console
class; - use of
java.util.Scanner
class.
The first approach is low-level and requires a large amount of "manual work", so now it is almost not
used. The second approach (class java.io.Console
, starting with JDK 1.6) also has certain
drawbacks,
in particular, the integrated environments Eclipse and IntelliJ IDEA do not support working with the objects of
these classes in their console windows.
The most convenient means of data input is a class java.util.Scanner
. The Scanner class provides
functions
for reading data from various sources, for example, from files. In our case, we indicate the need for reading
from
the keyboard (the standard input stream System.in
). The scanner object can be used to read
different
types of data. For example, the next()
function returns the next read value of the
String
type,
and the nextInt()
allows the integer to be obtained; nextDouble()
returns the read
double
.
There are also functions nextBoolean()
, nextByte()
, nextShort()
, nextLong()
,
nextFloat()
,
etc. All functions cause the program to stop executing, which is restarted after entering a corresponding value
from the keyboard.
To be able to work with the Scanner
class, the following import statement at the beginning of the
source code should be added:
import java.util.Scanner;
Now you can use the Scanner
class without any additional prefix.
First, you should create an object of this class using the new
operation (in detail this
operation
will be considered later). We connect the object-scanner with the standard System.in
stream. Then
you
can read different types of data. Example:
package ua.inf.iwanoff.java.first; import java.util.Scanner; public class ScannerTest { @SuppressWarnings("resource") public static void main(String[] args) { Scanner scanner = new Scanner(System.in); String s = scanner.next(); // reading string double d = scanner.nextDouble(); // reading floating point value int i = scanner.nextInt(); // reading integer value // ... use of read data } }
Note: The @SuppressWarnings("resource")
annotation is added so that the compiler
does not require closing the stream associated with the scanner
object. Standard console streams do
not require closing.
The great convenience of the class is the possibility of arbitrary location of data in the input stream:
separate
data can be divided by spaces, tabs, or new line symbols. The only exception is the use of the
nextLine()
function,
which reads the data to the end of line.
Output to the console window is done using the functions of the object out
of the
System
class.
The print()
function allows you to print the result without moving to a new line,
println()
moves
to a new line after the output.
You can also use the printf()
function for formatted output. The use of this method is similar to
the use of the corresponding function of the C language. The first parameter is the so-called formatting string.
You can then specify an arbitrary number of parameters whose values should be displayed on the console. Example:
double d = 3.5; int i = 12; System.out.printf("%f %d%n", d, i);
The following format specifiers are used:
Format specifier | Formatting |
---|---|
%a | Hexadecimal floating point value |
%b | Boolean value |
%c | Character |
%d | Decimal integer value |
%e | Decimal floating-point value in exponential (scientific) form |
%f | Decimal floating-point value in fixed-point form |
%g | %e or %f , whichever is shorter |
%o | Octal integer value |
%n | New line character |
%x | Hexadecimal integer value |
%% | % character |
After % character you can specify the width of the field.
There is also the ability to output messages to the System.err
stream (standard error message
stream).
Output to this stream has a higher priority than output to System.out
, so sometimes error messages
outperform the "normal" output.
2.6 Starting Java Application from the Command Line
Compiled Java programs can be launched from command line. There are different ways of getting command line in
different
operating systems. For instance, Windows provides cmd
command for this purpose. Within the command
line, you should launch Java virtual machine (java
command), followed by space character, followed
by -classpath
(or -cp
) parameter, followed by full path to project directory. Then you
should type name of a class with the main()
method. This name should be prefixed by package name.
Assume that project called First
was created in E:\workspace folder. To invoke main()
function
of TestClass
class, which allocated in first
package, you should enter the following
command:
java -cp E:\workspace\First first.TestClass
Sometimes you want to set particular code page, e.g. Cyrillic code page, for output. The command line argument
-Dfile.encoding=IBM-866
allows
you to set Cyrillic code page for DOS applications:
java -Dfile.encoding=IBM-866 -cp E:\workspace\First\bin first.TestClass
3 Sample Programs
3.1 Arithmetic Mean
Assume that we want to create a program that defines two variables and calculates their arithmetic mean (average).
A new project should be created. A new package called
ua.inf.iwanoff.java.first
will
contain the Average
class. Within class wizard window, generation of main()
function
should
be checked. The following code will be generated:
package ua.inf.iwanoff.java.first; public class Average { public static void main(String[] args) { } }
Now, instead of the comment inside the main()
function, we create an object of
the Scanner
class,
describe two variables, for example, a
and b
, read their values from the keyboard and
calculate the value of c
, which is their arithmetic mean.
Scanner s = new Scanner(System.in); double a = s.nextDouble(); int b = s.nextInt(); double c = (a + b) / 2;
To enable the use of the Scanner
class before the description of the Average
class,
we add an import statement (import java.util.Scanner;
). The definition
(description)
of a variable, as in C++, can be combined with the assignment of an initial value (initialization). In this
case,
we create a variable s
of type Scanner
, b
of type int
, and
also
variables a
and c
of type double
.
Necessary result soon calculated within initialization of c
variable. This
result
should be shown using System.out.println()
function.
System.out.println("Arithmetical mean: " + c);
The "plus" character in this line means concatenation of stings String type
constant "Arithmetic
mean: "
will be concatenated with string representation of c
.
The full text of our class will be as follows.
package ua.inf.iwanoff.java.first; import java.util.Scanner; public class Average { public static void main(String[] args) { Scanner s = new Scanner(System.in); double a = s.nextDouble(); int b = s.nextInt(); double c = (a + b) / 2; System.out.println("Arithmetic mean: " + c); } }
The result appears in the console view:
Arithmetical mean: 2.4
3.2 Powers of Two
Assume that we want to read n
(from 1 to 30) and print powers of 2, from the first
to the n
-th power. We can implement two approaches: using arithmetic operations and bitwise operations.
3.2.1 Use of Arithmetic Operations
A new class called Powers
can be added to previously created package. Our main()
function
will contain a single loop. Within its body, the next power of 2 should be calculated, based on previous one:
Scanner s = new Scanner(System.in); int n = s.nextInt(); int k = 2; // base of power int power = 1; for (int i = 1; i <= n; i++) { power *= k; System.out.printf("2 ^ %2d = %d\n", i, power); }
In this example, int
(integer) type is used to describe the variables, because
both the base of the degree, and the exponent, and the degree are integers. The initial value of the result
int power = 1;
is typical for computing products of sequences. The program can be as follows:
package ua.inf.iwanoff.java.first; import java.util.Scanner; public class Powers { public static void main(String[] args) { Scanner scanner = new Scanner(System.in); System.out.println("Enter n in the range from 1 to 30"); int n = scanner.nextInt(); int power = 1; final int k = 2; if (n < 0 || n > 30) { System.err.println("Wrong value of n"); } else { for (int i = 0; i <= n; i++) { System.out.printf("2 ^ %2d = %d\n", i, power); power *= k; } } } }
3.2.2 Use of Bitwise Operations
An approach that uses a bitwise shift operation will be more efficient.
package ua.inf.iwanoff.java.first; import java.util.Scanner; public class PowersOfTwo { public static void main(String[] args) { Scanner scanner = new Scanner(System.in); System.out.println("Enter n in the range from 1 to 30"); int n = scanner.nextInt(); if (n < 0 || n > 30) { System.err.println("Wrong value of n"); } else { for (int i = 0; i <= n; i++) { System.out.printf("2 ^ %2d = %d\n", i, 1 << i); } } } }
3.3 The Greatest Common Divisor
Suppose you want to calculate the greatest common divisor. We'll use of the Euclidean algorithm, which assumes subtraction of smaller number from larger one and replacement of larger number with resulting difference. The algorithm ends when the two numbers are equal. It will look like this:
package ua.inf.iwanoff.java.first; import java.util.Scanner; public class GreatestCommonDivisor { static int gcd(int m, int n) { while (m != n) { if (m > n) m -= n; else n -= m; } return m; } public static void main(String[] args) { Scanner s = new Scanner(System.in); int m = s.nextInt(); int n = s.nextInt(); System.out.println(gcd(m, n)); } }
The result can be used to calculate the lowest common multiple of numbers. To do this, their product should be divided by the greatest common divisor.
3.4 Recursion
Suppose we need to create a recursive function to calculate the integer power of a real number and perform testing for different values. The function is placed in a separate class:
package ua.inf.iwanoff.java.first; public class IntPower { public static double power(double x, int n) { if (n < 0) { return 1 / power(x, -n); } if (n == 0) { return 1; } return x * power(x, n - 1); } }
In another class, we can use static import. The Program
class will look like this:
package uua.inf.iwanoff.java.first; import java.util.Scanner; import static ua.inf.iwanoff.java.first.IntPower.*; public class Program { @SuppressWarnings("resource") public static void main(String[] args) { Scanner scanner = new Scanner(System.in); System.out.print("Enter x: "); double x = scanner.nextDouble(); System.out.print("Enter n: "); int n = scanner.nextInt(); double p = power(x, n); System.out.println("Power: " + p); } }
3.5 Calculation of an Expression
In the next program we'll read from the command line value of x and calculate y using the following formula:
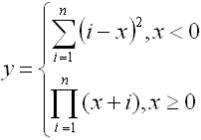
when n is equal to 6. Calculation of y should be implemented in a separate function. The program can be as follows:
package ua.inf.iwanoff.java.first; import java.util.Scanner; public class Formula { public static double f(double x) { final int n = 6; double y; if (x < 0) { y = 0; for (int i = 1; i <= n; i++) { y += (i - x) * (i - x); } } else { y = 1; for (int i = 1; i <= n; i++) { y *= (x + i); } } return y; } public static void main(String[] args) { Scanner s = new Scanner(System.in); double x = s.nextDouble(); double y = f(x); // Invocation of a function System.out.println("x = " + x + "\ty = " + y); } }
3.6 Table of Square Roots
The following program reads interval boundaries and step of argument increasing from the keyboard and prints in a cycle values of square roots of intermediate values:
package ua.inf.iwanoff.java.first; import java.util.Scanner; public class SquareRoots { public static void main(String[] args) { Scanner s = new Scanner(System.in); double from = s.nextDouble(); double to = s.nextDouble(); double step = s.nextDouble(); if (from < 0 || to < 0 || from >= to || step <= 0) { System.out.println("Wrong data!"); } else { // The main program loop: for (double x = from; x <= to; x += step) { System.out.println("x = " + x + "\t y = " + Math.sqrt(x)); } } } }
The table of x and y values should be shown after launching program.
4 Exercises
- Enter two valid numbers. Find and display the arithmetic mean of the squares of numbers.
- Enter two valid numbers. Find and display geometric mean - square root of their product. If the root is not possible to calculate, display an error message.
- Enter a positive integer. Check whether it is prime number. Output under "yes" or "no".
- Enter a positive integer. Check whether it is an exact square of another integer. Print the number found, or "no" otherwise.
- Enter two integer positive numbers. Find and display the lowest common multiple of these numbers.
- Create a recursive function for calculating the sum of squares of the first n numbers.
- Create a recursive function for calculating the product of the sines of the first n natural numbers.
5 Quiz
- What is Java platform?
- What is the difference between JDK and JRE?
- What is Java Virtual Machine?
- What is byte code?
- Which files contain Java source code and bytecodes?
- When and how to link and build a Java application?
- What are advantages of Java programming languages (in comparison with C++)?
- What is the difference between keywords and reserved words?
- What is Javadoc comment?
- How to create built-in documentation?
- What is the difference between Java fundamental data types and ones in C++?
- What is the usage of control sequences?
- How to implement type cast?
- What is special in
boolean
type? - How to cast
boolean
value to the integer type? - Can you apply
&
operator instead of&&
operator to operands ofboolean
type? - Is
break
statement always needing label? - Is it possible to put multiple packages in the same source file?
- Can you access names defined in some another package without
import
statement? - What are the advantages and disadvantages of the static import?